Introduction
Web development is a dynamic field that constantly evolves as new technologies, frameworks, and best practices emerge. Whether you’re a seasoned developer or just starting, there’s always something new to learn. This blog post will dive into some of the most effective web development tips and tricks to help you build better, faster, and more efficient websites. From optimizing performance to writing clean code, this guide has it all.
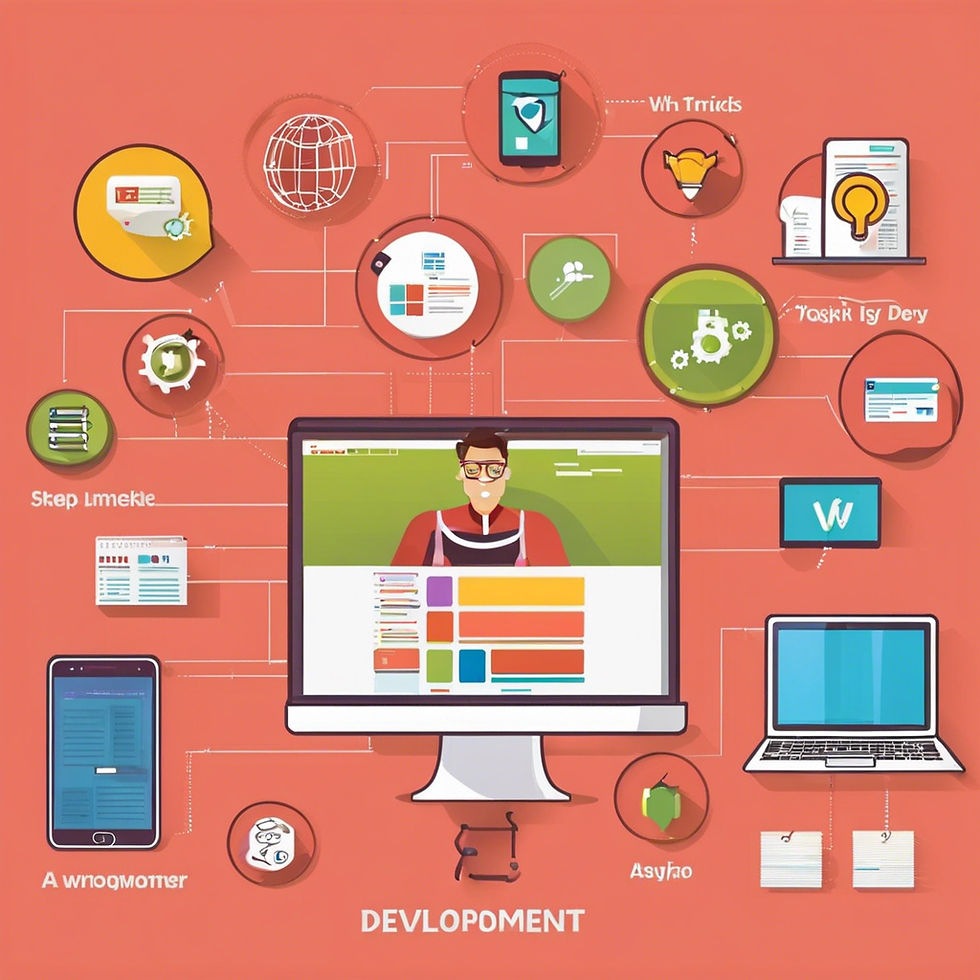
1. Prioritize Performance Optimization
Importance of Website Performance
Performance optimization should be at the forefront of your web development process. A fast-loading website not only provides a better user experience but also positively impacts your SEO rankings. Users expect websites to load within a few seconds, and even a slight delay can lead to increased bounce rates.
Practical Tips for Performance Optimization
1. Minimize HTTP Requests: Reduce the number of elements on your page (images, scripts, CSS files) that require HTTP requests. Combining CSS and JavaScript files can significantly reduce these requests.
2. Use a Content Delivery Network (CDN): CDNs distribute your content across multiple servers worldwide, ensuring faster content delivery by serving it from the nearest location to the user.
3. Optimize Images: Use modern image formats like WebP, which offer better compression than JPEG or PNG. Also, utilize image optimization tools like ImageMagick or TinyPNG to reduce file sizes.
4. Leverage Browser Caching: By enabling browser caching, you allow static resources to be stored locally on a user’s device, reducing load times on subsequent visits.
5. Lazy Loading Images: Implement lazy loading for images that are not immediately visible on the user’s screen. This technique delays loading images until they’re needed, speeding up initial page load times.
Example
<img src="image.webp" loading="lazy" alt="Optimized Image">
Using the loading="lazy" attribute in your image tags is an easy way to implement lazy loading.
2. Write Clean and Maintainable Code
Importance of Code Quality
Writing clean, readable, and maintainable code is crucial for long-term success in web development. It reduces the likelihood of bugs, makes collaboration easier, and ensures that future updates or changes can be made with minimal headaches.
Practical Tips for Writing Clean Code
1. Use Meaningful Variable and Function Names: Avoid generic names like data or temp. Instead, use descriptive names that explain the purpose of the variable or function.
2. Keep Functions Small and Focused: A function should do one thing and do it well. Breaking down large functions into smaller, reusable ones improves readability and makes your code easier to debug.
3. Comment Your Code Wisely: While commenting is important, avoid over-commenting. Focus on explaining the “why” rather than the “what” if the code is self-explanatory.
4. Follow a Consistent Coding Style: Whether it’s using camelCase or snake_case for variable names, consistency is key. Many teams adopt style guides like Airbnb’s JavaScript style guide to ensure uniformity.
5. Refactor Regularly: Regularly revisiting and refining your code can eliminate redundancy, improve performance, and ensure that your codebase stays clean over time.
Example
// BAD
function processData() {
let x = document.getElementById('data').value;
let y = x.split(' ');
let z = y.map(a => a.trim());
return z;
}
// GOOD
function getTrimmedWords(data) {
return data.split(' ').map(word => word.trim());
}
In the above example, the improved function name and refactored code make it easier to understand and maintain.
3. Master Responsive Design
Importance of Responsive Design
With the increasing use of mobile devices, ensuring that your website looks and functions well on all screen sizes is essential. Responsive design allows your site to adapt to different devices, providing a seamless user experience regardless of screen size.
Practical Tips for Responsive Design
1. Use Flexible Grids and Layouts: CSS Grid and Flexbox are powerful tools for creating responsive layouts. They allow you to create flexible designs that adjust to different screen sizes.
2. Set Breakpoints Thoughtfully: Choose breakpoints based on your content and design rather than specific devices. This approach ensures that your design looks good on a wide range of devices.
3. Responsive Typography: Use relative units like em or rem for font sizes instead of pixels. This makes your text more adaptable to different screen sizes and user preferences.
4. Optimize Media Queries: Media queries allow you to apply CSS rules based on the device’s characteristics, such as width or height. Use them to adjust layouts, font sizes, and other design elements for different screen sizes.
5. Test Across Multiple Devices: Regularly test your website on different devices and screen sizes to ensure consistency. Tools like BrowserStack or real device labs can help with this.
Example
/* Responsive Grid Layout */
.container {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(250px, 1fr));
gap: 20px;
}
/* Media Query for Mobile Devices */
@media (max-width: 600px) {
.container {
grid-template-columns: 1fr;
}
}
This CSS snippet creates a responsive grid layout that adjusts based on the screen size.
4. Enhance User Experience (UX) with Thoughtful Design
Importance of UX
User experience (UX) is a critical aspect of web development that directly impacts user satisfaction and retention. A well-designed website that is easy to navigate and visually appealing will keep users engaged and encourage them to return.
Practical Tips for Enhancing UX
1. Keep Navigation Simple: Ensure that users can easily find what they’re looking for. Use clear labels for navigation links and keep the structure intuitive.
2. Improve Readability: Choose fonts that are easy to read and ensure sufficient contrast between text and background. Break up large blocks of text with headings, bullet points, and images.
3. Use Visual Hierarchy: Guide users’ attention by emphasizing important elements like calls to action (CTAs). Use size, color, and positioning to create a visual hierarchy.
4. Optimize Forms: Keep forms simple by asking only for necessary information. Use placeholder text and clear labels to guide users through the process.
5. Implement Accessibility Features: Make your website accessible to all users, including those with disabilities. Use semantic HTML, provide alt text for images, and ensure that your site is navigable via keyboard.
Example
<!-- Accessible Form -->
<form aria-label="Contact Form">
<label for="name">Name</label>
<input type="text" id="name" name="name" required>
<label for="email">Email</label>
<input type="email" id="email" name="email" required>
<button type="submit">Submit</button>
</form>
The above form uses the aria-label attribute to make it accessible for screen readers, improving the user experience for all visitors.
5. Stay Up-to-Date with JavaScript Best Practices
Importance of JavaScript in Web Development
JavaScript is the backbone of modern web development, powering everything from simple animations to complex web applications. Staying up-to-date with the latest best practices ensures that your JavaScript code is efficient, secure, and maintainable.
Practical Tips for Writing Better JavaScript
1. Use Modern ES6+ Syntax: Take advantage of the features introduced in ECMAScript 6 and beyond, such as let, const, arrow functions, template literals, and destructuring.
2. Avoid Global Variables: Minimize the use of global variables to reduce the risk of conflicts and bugs. Encapsulate code within functions or modules.
3. Debounce and Throttle Events: When handling events like scrolling or resizing, use debounce or throttle techniques to limit the frequency of function calls, improving performance.
4. Use Promises and Async/Await for Asynchronous Code: Handling asynchronous operations is easier and more readable with Promises or async/await syntax, compared to traditional callbacks.
5. Implement Error Handling: Always include error handling in your code, especially for asynchronous operations. Use try...catch blocks or handle rejections in Promises.
Example
// Async/Await Example with Error Handling
async function fetchData(url) {
try {
let response = await fetch(url);
if (!response.ok) {
throw new Error('Network response was not ok');
}
let data = await response.json();
return data;
} catch (error) {
console.error('There has been a problem with your fetch operation:', error);
}
}
This example demonstrates how to use async/await for fetching data and includes error handling to manage potential issues.
6. Optimize for SEO and Web Accessibility
Importance of SEO and Accessibility
Search engine optimization (SEO) and web accessibility are critical factors in ensuring that your website reaches the widest possible audience. SEO helps your site rank higher in search engine results, driving more organic traffic, while accessibility ensures that all users, including those with disabilities, can access and navigate your site effectively.
Practical Tips for SEO Optimization
1. Use Semantic HTML: Semantic HTML elements like <header>, <nav>, <article>, and <footer> not only improve the accessibility of your site but also help search engines better understand your content structure.
2. Optimize Meta Tags: Ensure that each page has a unique and descriptive title tag and meta description. Use important keywords naturally within these tags to improve your site’s SEO.
3. Create High-Quality Content: Content is king when it comes to SEO. Focus on creating valuable, relevant, and original content that answers your audience’s questions or solves their problems. Incorporate important keywords, but avoid keyword stuffing.
4. Optimize Images with Alt Text: Use descriptive alt text for images. This not only helps with accessibility but also allows search engines to index your images, contributing to your site’s SEO.
5. Improve Site Speed: As discussed earlier, site speed is a significant ranking factor in SEO. Use tools like Google PageSpeed Insights to identify and fix issues that may be slowing down your site.
Practical Tips for Web Accessibility
1. Use ARIA Attributes: Accessible Rich Internet Applications (ARIA) attributes help improve accessibility by providing additional context to screen readers. Use ARIA roles, properties, and states where necessary.
2. Provide Keyboard Navigation: Ensure that all interactive elements, such as buttons, links, and forms, are accessible via keyboard navigation. This is crucial for users who rely on keyboards or assistive technologies.
3. Ensure Color Contrast: Use tools like the WebAIM Contrast Checker to ensure sufficient contrast between text and background colors, making your content readable for all users, including those with visual impairments.
4. Implement Skip Navigation Links: Include a “Skip to content” link at the top of your pages, allowing keyboard users to bypass repetitive navigation links and go directly to the main content.
5. Test Accessibility Regularly: Use accessibility testing tools like WAVE or Axe to evaluate your site’s accessibility. Regular testing ensures that your site meets accessibility standards and provides a positive user experience for everyone.
Example
<!-- Example of Semantic HTML and ARIA Attributes -->
<header>
<h1>Web Development Tips and Tricks</h1>
<nav aria-label="Main navigation">
<ul>
<li><a href="#performance">Performance Optimization</a></li>
<li><a href="#clean-code">Clean Code</a></li>
<li><a href="#responsive-design">Responsive Design</a></li>
<li><a href="#ux">User Experience</a></li>
<li><a href="#javascript">JavaScript Best Practices</a></li>
<li><a href="#seo-accessibility">SEO & Accessibility</a></li>
</ul>
</nav>
</header>
In this example, the navigation uses an aria-label attribute for better accessibility, and the HTML structure is semantic, aiding both SEO and accessibility.
7. Version Control Best Practices
Importance of Version Control
Version control systems like Git are essential for managing changes to your codebase. They allow you to track revisions, collaborate with others, and maintain a history of your project’s development. Following best practices in version control ensures that your project remains organized and that team collaboration runs smoothly.
Practical Tips for Version Control
1. Use Meaningful Commit Messages: Write clear and concise commit messages that describe the changes made. This makes it easier to understand the history of the project when reviewing commits.
2. Branching Strategy: Implement a branching strategy that works for your team. Common strategies include Git Flow, GitHub Flow, and trunk-based development. Using branches for new features, bug fixes, and experiments keeps the main codebase stable.
3. Regular Commits: Commit changes frequently to avoid losing work and to keep the history of changes granular. This also makes it easier to isolate issues when debugging.
4. Code Reviews: Implement code reviews as part of your version control workflow. Code reviews help catch errors, improve code quality, and share knowledge within the team.
5. Use Tags for Releases: Use Git tags to mark important points in your project, such as releases or major updates. Tags make it easier to reference and deploy specific versions of your application.
Example
# Example of creating a new branch and committing changes
git checkout -b feature-responsive-layout
# Make your changes in the codebase
git add .
git commit -m "Implemented responsive layout for homepage"
git push origin feature-responsive-layout
In this example, a new branch is created for a responsive layout feature, and changes are committed with a descriptive message.
8. Security Best Practices
Importance of Security in Web Development
Security is a critical aspect of web development that cannot be overlooked. Ensuring that your website or application is secure protects sensitive data, maintains user trust, and complies with legal requirements. Security breaches can have severe consequences, including data loss, financial loss, and reputational damage.
Practical Tips for Web Security
1. Use HTTPS: Secure your site with HTTPS to encrypt data transmitted between the user and the server. Obtain an SSL/TLS certificate and configure your server to redirect all HTTP traffic to HTTPS.
2. Sanitize User Input: Prevent SQL injection, cross-site scripting (XSS), and other attacks by sanitizing and validating user input. Use prepared statements in SQL queries and encode output to prevent script injection.
3. Implement Strong Authentication: Use strong passwords, multi-factor authentication (MFA), and secure password storage methods (such as bcrypt) to protect user accounts.
4. Keep Software Updated: Regularly update your server, CMS, libraries, and dependencies to patch known vulnerabilities. Automated tools like Dependabot can help manage dependencies and alert you to updates.
5. Regular Security Audits: Conduct regular security audits and penetration testing to identify and fix vulnerabilities in your application. Tools like OWASP ZAP or Burp Suite can assist in these audits.
Example
# Example of generating a bcrypt hash for password storage in Node.js
const bcrypt = require('bcrypt');
const saltRounds = 10;
const password = 'userPassword123';
bcrypt.hash(password, saltRounds, function(err, hash) {
if (err) {
console.error('Error hashing password:', err);
} else {
console.log('Hashed password:', hash);
// Store the hash in your database
}
});
This Node.js example demonstrates how to use bcrypt to hash a password before storing it, enhancing security for user authentication.
9. Effective Debugging Techniques
Importance of Debugging
Debugging is an essential skill for web developers. No matter how experienced you are, bugs and errors are inevitable. Being able to efficiently identify and fix issues in your code ensures that your projects run smoothly and are delivered on time.
Practical Tips for Debugging
1. Use Console Logs Strategically: While console.log is a simple but effective debugging tool, use it strategically. Log important variables or data points rather than cluttering the console with excessive logs.
2. Leverage Debugging Tools: Modern browsers like Chrome and Firefox offer powerful debugging tools. Use breakpoints, watch variables, and step through code to understand the flow and catch bugs.
3. Read Error Messages Carefully: Error messages often provide valuable clues about what went wrong. Pay attention to the details in the message and trace back to the source of the issue.
4. Isolate the Problem: Break down the code to isolate the section where the problem occurs. By narrowing down the focus, you can more easily identify the root cause.
5. Peer Reviews: Sometimes a fresh pair of eyes can spot issues that you might have missed. Involve a colleague in the debugging process for a different perspective.
Example
// Using breakpoints in Chrome DevTools for debugging
function calculateTotal(items) {
let total = 0;
items.forEach(item => {
total += item.price * item.quantity;
// Set a breakpoint here to inspect 'total' and 'item'
});
return total;
}
// Test case
const cartItems = [
{ price: 100, quantity: 2 },
{ price: 200, quantity: 1 }
];
console.log('Total:', calculateTotal(cartItems));
In this example, you can use a breakpoint within the loop to inspect variables and understand how the calculation is performed.
10. Continuous Learning and Staying Updated
Importance of Lifelong Learning
The web development landscape is constantly evolving, with new technologies, frameworks, and best practices emerging regularly. To stay competitive and efficient, it’s essential to commit to continuous learning and keep your skills up-to-date.
Practical Tips for Continuous Learning
1. Follow Industry Leaders: Stay informed by following industry leaders, blogs, and podcasts. Platforms like Twitter, LinkedIn, and GitHub are great for keeping up with the latest trends and insights.
2. Experiment with New Technologies: Set aside time to experiment with new tools, libraries, and frameworks. Building small side projects is a great way to learn and apply new skills.
3. Participate in Online Communities: Engage with online communities like Stack Overflow, Reddit, or web development forums. These platforms provide valuable opportunities to learn from others and share your knowledge.
4. Take Online Courses: Invest in your education by taking online courses on platforms like Udemy, Coursera, or freeCodeCamp. These courses often offer structured learning paths and hands-on projects that can help you master new skills quickly.
5. Attend Conferences and Meetups: Web development conferences and local meetups are excellent opportunities to learn from experts, network with peers, and stay updated on the latest industry trends. Many conferences also offer online streaming options, making it easier to participate.
Example
// Example of exploring a new JavaScript library
import axios from 'axios';
async function fetchData(url) {
try {
const response = await axios.get(url);
console.log('Data:', response.data);
} catch (error) {
console.error('Error fetching data:', error);
}
}
// Test case
fetchData('https://api.example.com/data');
In this example, experimenting with the Axios library for making HTTP requests provides an opportunity to learn and understand its advantages over native fetch in certain scenarios.
11. Effective Collaboration in Web Development
Importance of Collaboration
Web development is rarely a solo endeavor. Whether you’re working within a team or collaborating with clients, effective communication and collaboration are key to successful project outcomes. Leveraging collaborative tools and practices can streamline the development process and improve the quality of the final product.
Practical Tips for Collaboration
1. Use Version Control Effectively: As discussed earlier, version control systems like Git are essential for collaborative projects. Ensure that everyone on the team follows the same branching strategy and commit practices.
2. Leverage Project Management Tools: Tools like Jira, Trello, or Asana can help manage tasks, track progress, and ensure that everyone on the team is aligned. These tools allow for better planning, clear communication, and easy tracking of deliverables.
3. Conduct Regular Standups: Daily or weekly standup meetings help keep the team on the same page. Use these meetings to discuss what was accomplished, what is being worked on, and any blockers that need addressing.
4. Utilize Code Collaboration Platforms: Platforms like GitHub and GitLab offer collaborative features such as pull requests and code reviews. These allow team members to contribute code, review each other’s work, and maintain a high standard of code quality.
5. Encourage Open Communication: Create an environment where team members feel comfortable sharing their ideas, asking questions, and providing feedback. Tools like Slack or Microsoft Teams can facilitate open communication, especially in remote teams.
6. Document Everything: Maintain clear and comprehensive documentation for all aspects of your project, including code, APIs, and processes. Good documentation reduces confusion, aids onboarding new team members, and ensures that knowledge is easily accessible.
Example
# Example of a Pull Request Description
## Summary
This pull request implements a responsive layout for the homepage, using CSS Grid and Flexbox. It addresses issue #45.
## Changes
- Updated `index.html` with new container classes.
- Added responsive CSS rules in `styles.css`.
- Refactored JavaScript to handle dynamic content resizing.
## Testing
- Tested on Chrome, Firefox, and Safari.
- Verified responsiveness on mobile, tablet, and desktop screen sizes.
## Notes
- Need feedback on the grid spacing between elements.
- Will add unit tests for the new JavaScript functions in a separate PR.
In this example, a detailed pull request description helps team members understand the changes, testing performed, and areas where feedback is needed, promoting effective collaboration.
12. Embrace Automation in Web Development
Importance of Automation
Automation can significantly increase productivity, reduce errors, and ensure consistency across your web development projects. By automating repetitive tasks, you can focus on more complex aspects of development and improve the overall efficiency of your workflow.
Practical Tips for Automation
1. Use Task Runners and Build Tools: Tools like Gulp, Grunt, and Webpack automate tasks such as minification, compilation, linting, and image optimization. These tools ensure that your code is production-ready with minimal manual effort.
2. Automate Testing: Implement automated testing using frameworks like Jest, Mocha, or Cypress. Automated tests ensure that your application functions as expected and reduces the likelihood of bugs being introduced during development.
3. Continuous Integration and Continuous Deployment (CI/CD): Set up CI/CD pipelines with tools like Jenkins, Travis CI, or GitHub Actions. These pipelines automatically build, test, and deploy your code every time changes are made, ensuring that your application is always in a deployable state.
4. Automate Code Formatting: Use tools like Prettier or ESLint to enforce consistent code formatting across your project. These tools can be integrated into your CI pipeline or used as pre-commit hooks to automatically format code before it’s committed.
5. Leverage DevOps Practices: Automating infrastructure management using tools like Docker, Kubernetes, or Terraform can streamline the deployment process, making it easier to scale applications and manage complex environments.
Example
# Example of a GitHub Actions CI Workflow
name: CI Workflow
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
- name: Build project
run: npm run build
This example demonstrates a simple CI pipeline using GitHub Actions to automatically run tests and build the project whenever changes are pushed to the main branch.
13. Focus on Scalability from the Start
Importance of Scalability
Scalability is the ability of your web application to handle increased traffic and data without compromising performance. Designing for scalability from the start ensures that your application can grow with your user base and continue to perform well under heavy loads.
Practical Tips for Building Scalable Applications
1. Design a Scalable Architecture: Use microservices architecture to break down your application into smaller, independent services that can be scaled individually. This approach improves flexibility and allows you to optimize resources more effectively.
2. Optimize Database Performance: Use indexing, query optimization, and caching strategies to improve database performance. Consider using NoSQL databases like MongoDB or distributed databases like Cassandra for handling large-scale data.
3. Implement Load Balancing: Use load balancers to distribute incoming traffic across multiple servers, ensuring that no single server becomes a bottleneck. This also improves the reliability and availability of your application.
4. Use Cloud Services: Cloud platforms like AWS, Azure, or Google Cloud offer scalable infrastructure and services that can grow with your application. Take advantage of auto-scaling features to automatically adjust resources based on demand.
5. Monitor and Optimize Performance: Regularly monitor your application’s performance and make adjustments as needed. Tools like New Relic, Datadog, or AWS CloudWatch can provide insights into performance bottlenecks and help you optimize resource usage.
Example
// Example of Implementing Caching with Redis in Node.js
const redis = require('redis');
const client = redis.createClient();
function cacheMiddleware(req, res, next) {
const { id } = req.params;
client.get(id, (err, data) => {
if (err) throw err;
if (data !== null) {
res.send(JSON.parse(data));
} else {
next();
}
});
}
app.get('/api/data/:id', cacheMiddleware, (req, res) => {
const data = fetchDataFromDatabase(req.params.id);
client.setex(req.params.id, 3600, JSON.stringify(data)); // Cache for 1 hour
res.send(data);
});
In this example, a Redis cache is used to store database query results, reducing load times and improving scalability by minimizing the need to repeatedly query the database.
14. Prioritize Mobile-First Design
Importance of Mobile-First Design
With the increasing use of mobile devices to access the web, adopting a mobile-first design approach is essential. Designing for mobile devices first ensures that your website provides a seamless experience for the majority of users, and then you can progressively enhance the experience for larger screens.
Practical Tips for Mobile-First Design
1. Simplify the Layout: Start with a simple layout that works well on small screens. Focus on essential content and navigation elements, and remove any unnecessary clutter.
2. Optimize Touch Interactions: Ensure that buttons and links are large enough to be easily tapped with a finger. Provide sufficient spacing between interactive elements to avoid accidental clicks.
3. Use Responsive Images: Serve appropriately sized images based on the user’s device. Use the srcset attribute to define different image sources for different screen resolutions.
4. Prioritize Performance on Mobile: Mobile devices often have slower internet connections and less processing power than desktops. Optimize your site for speed by minimizing resource use and enabling compression.
5. Test on Real Devices: Regularly test your mobile-first design on various devices to ensure it performs well across different screen sizes and orientations.
Example
<!-- Example of Responsive Images with srcset -->
<img src="image-small.jpg"
srcset="image-small.jpg 480w, image-medium.jpg 800w, image-large.jpg 1200w"
sizes="(max-width: 600px) 480px, (max-width: 1200px) 800px, 1200px"
alt="Responsive Image">
In this example, the srcset attribute is used to serve different image sizes based on the screen width, optimizing performance and user experience on mobile devices.
15. Embrace Progressive Web Apps (PWAs)
Importance of PWAs
Progressive Web Apps (PWAs) offer the best of both web and mobile apps, providing a fast, reliable, and engaging user experience. PWAs can work offline, load quickly, and provide native-like interactions, making them an excellent choice for modern web development.
Practical Tips for Building PWAs
1. Use Service Workers: Service workers are the backbone of PWAs, enabling offline functionality and background synchronization. Implement service workers to cache assets and handle network requests even when the user is offline.
2. Implement a Web App Manifest: The web app manifest provides metadata about your app, such as the name, icon, and theme color. This allows users to add your PWA to their home screen with a custom icon and splash screen.
3. Ensure Fast Loading Times: Optimize your PWA for speed by minimizing resource use, leveraging lazy loading, and using efficient caching strategies. Fast loading times are crucial for providing a seamless user experience.
4. Enable Push Notifications: PWAs can send push notifications to re-engage users. Implement push notifications to keep users informed and encourage them to return to your app.
5. Test PWA Performance: Use tools like Lighthouse to audit your PWA and ensure it meets performance, accessibility, and best practice standards.
Example
// Example of Registering a Service Worker for a PWA
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('/service-worker.js')
.then(registration => {
console.log('Service Worker registered with scope:', registration.scope);
})
.catch(error => {
console.error('Service Worker registration failed:', error);
});
}
// service-worker.js
self.addEventListener('install', event => {
event.waitUntil(
caches.open('v1').then(cache => {
return cache.addAll([
'/',
'/index.html',
'/styles.css',
'/app.js',
'/images/icon.png',
]);
})
);
});
self.addEventListener('fetch', event => {
event.respondWith(
caches.match(event.request).then(response => {
return response || fetch(event.request);
})
);
});
In this example, a service worker is registered and configured to cache essential assets, enabling offline functionality and faster load times for repeat visits, which are key characteristics of a Progressive Web App.
Conclusion
Web development is a multifaceted discipline that requires continuous learning, adaptability, and a keen eye for detail. By implementing the tips and tricks covered in this guide, you can enhance your skills, improve the quality of your work, and stay ahead in the ever-evolving field of web development.
From optimizing performance and embracing responsive design to mastering JavaScript best practices and securing your web applications, each tip contributes to building better, more efficient, and user-friendly websites. Whether you’re a beginner or a seasoned developer, these strategies will help you deliver robust, high-quality web experiences that meet the demands of modern users.
Keep experimenting, stay curious, and never stop learning. The web development landscape is always changing, and your ability to adapt and grow with it will be key to your success.
Комментарии